A Beginner's Guide to Integrating Bootstrap with React
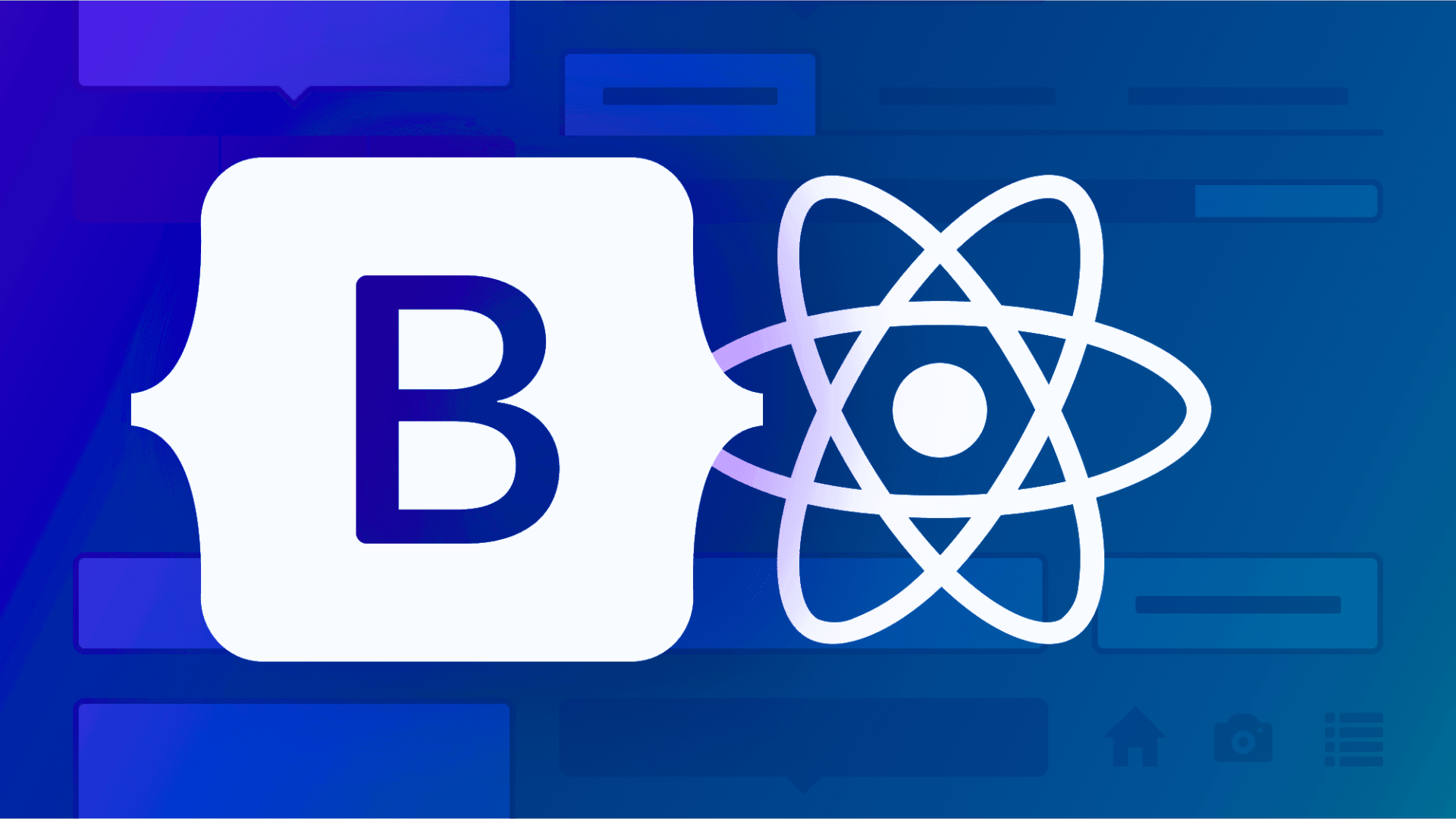
Creating responsive, visually appealing web applications is essential for any developer, and combining the power of React with the simplicity of Bootstrap is an effective way to achieve this. In this guide, we’ll walk you through how to easily integrate Bootstrap into your React projects, enabling you to build beautiful, responsive interfaces quickly and efficiently.Whether you’re new to React, Bootstrap, or both, this guide from Aryu Academy will provide you with a solid foundation.
What is Bootstrap?
Bootstrap is a popular CSS framework that simplifies web design with prebuilt components and styles for typography, forms, buttons, navigation, and more. It’s especially useful for creating responsive designs that look great on any device, which is why it’s often paired with React for fast and attractive front-end development.
Why Use Bootstrap with React?
Combining Bootstrap with React offers the best of both worlds:
- Speed: With prebuilt components, you can skip repetitive styling.
- Responsiveness: Bootstrap’s grid system is built to be responsive, making it easier to design for mobile and desktop views.
- Consistency: Bootstrap maintains a consistent look and feel across different components, making your app look polished.
Getting Started: Adding Bootstrap to Your React Project
Let’s dive into how you can set up Bootstrap with React.
Step 1: Setting Up Your React Project
“For those without an existing React project, start by setting one up with Create React App:”
bash
npx create-react-app my-bootstrap-app
cd my-bootstrap-app
This command will create a new React project in the my-bootstrap-app folder and set up all necessary dependencies.
Step 2: Installing Bootstrap
There are two main ways to integrate Bootstrap with React:
- Using Bootstrap CDN: For a quick start, you can add a link to Bootstrap’s CSS in your public/index.html file.
html
<!– Add this line in the <head> section of public/index.html –>
<link rel=”stylesheet”
href=”https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstra
p.min.css”> - Installing Bootstrap via npm: If you want more control over your Bootstrap files and to manage dependencies better, it’s recommended to install Bootstrap via npm.
bash
npm install bootstrap
After installing, import Bootstrap’s CSS file in your main index.js file:
javascript
Copy code
import ‘bootstrap/dist/css/bootstrap.min.css’;
Step 3: Using Bootstrap Components in React
With Bootstrap installed, you can start using its classes directly in your JSX files.
javascript
// ExampleComponent.js
import React from ‘react’;
const ExampleComponent = () => {
return (
<div className=”container mt-5″>
<h1 className=”text-center text-primary”>Welcome to My Bootstrap-React App</h1>
<p className=”lead text-center”>
This is a simple example of using Bootstrap with React.
</p>
<div className=”d-flex justify-content-center”>
<button className=”btn btn-primary”>Click Me</button>
</div>
</div>
);
};
export default ExampleComponent;
In this code, we use the container, text-center, text-primary, lead, and btn classes from Bootstrap to style our component.
Step 4: Working with Bootstrap’s Grid System in React
“Bootstrap’s grid system is an essential tool for building responsive layouts effortlessly.“. Here’s how you can use it with React :
javascript
// GridExample.js
import React from ‘react’;
const GridExample = () => {
return (
<div className=”container”>
<div className=”row”>
<div className=”col-md-4″>Column 1</div>
<div className=”col-md-4″>Column 2</div>
<div className=”col-md-4″>Column 3</div>
</div>
</div>
);
};
export default GridExample;
This code will create a responsive layout with three columns, where each column takes up 1/3 of the container on medium screens and above.
Advanced Tip: Using React-Bootstrap
While the basic Bootstrap library works well with React, React-Bootstrap provides prebuilt React components for Bootstrap elements, which simplifies things even further. You can install it with:
bash
npm install react-bootstrap
With React-Bootstrap, you can use components like Button, Card, and Modal without needing to write custom JSX for each. Here’s an example:
javascript
// ReactBootstrapExample.js
import React from ‘react’;
import { Button, Card } from ‘react-bootstrap’;
const ReactBootstrapExample = () => {
return (
<div className=”d-flex justify-content-center mt-5″>
<Card style={{ width: ’18rem’ }}>
<Card.Body>
<Card.Title>React-Bootstrap Card</Card.Title>
<Card.Text>
This card uses React-Bootstrap components for a consistent,styled look.
</Card.Text>
<Button variant=”primary”>Learn More</Button>
</Card.Body>
</Card>
</div>
);
};
export default ReactBootstrapExample;
Summary
Integrating Bootstrap with React is a powerful way to create polished, responsive applications quickly. This approach keeps your code organized and lets you leverage pre-styled, mobile-friendly components. Whether you prefer traditional Bootstrap or React-Bootstrap, you now have the tools to start styling your React apps with ease.
At Aryu Academy, we believe in empowering beginners and experienced developers alike with the knowledge to build high-quality web applications. Ready to dive deeper? Enroll in our web development courses today to unlock the full potential of front-end frameworks and libraries
Recent Posts
The Evolution of UI/UX Design: Key Milestones and Innovations User Interface (UI) and User Experience (UX) design have become essential […]
Social Media Marketing vs. SEO: Which Strategy Works Best for Your Business? For businesses today, online presence is essential. But […]
The Importance of Backlinks in SEO: Building Your Authority In today’s digital landscape, SEO (Search Engine Optimization) is vital to […]
A Beginner’s Guide to Integrating Bootstrap with React Creating responsive, visually appealing web applications is essential for any developer, and […]